14. Java Object Serialization
Java Object Serialization
In this section, you will learn how to use Java's built-in serialization mechanism.
ND079 JPND C2 L02 A15 Serializing Data In Java
What is Serialization?
Serialization is the process of converting an object into a data format that can later be deserialized back into the original object.
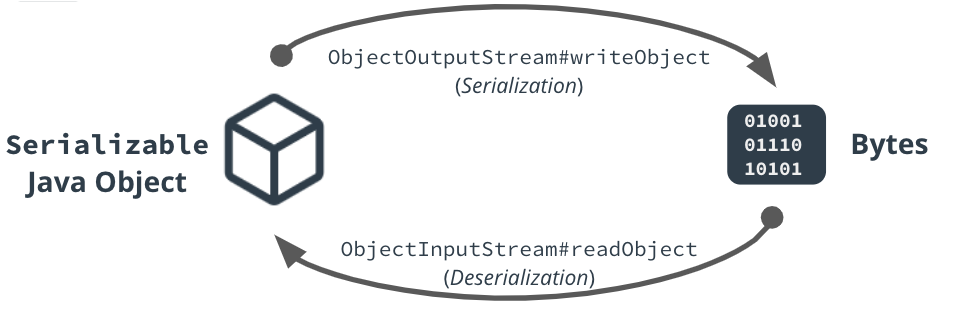
Java Object Serialization
To serialize the Java object, create an ObjectOutputStream
, and pass the object to the streams writeObject()
method. The ObjectOutputStream
writes a sequence of bytes, and its constructor takes another stream as an argument.
To recreate the original Java object from the serialized bytes, read the bytes back into a ObjectInputStream
.
Example
Here's a really bare-bones example demonstrating the basic procedure to serialize and deserialize a Java object:
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
public final class Main {
public static void main(String[] args) throws Exception {
Path path = Path.of("list.bin");
try (var out = new ObjectOutputStream(Files.newOutputStream(path))) {
out.writeObject(List.of("Hello", " ", "World!"));
}
try (var in = new ObjectInputStream(Files.newInputStream(path))) {
List<String> deserialized = (List<String>) in.readObject();
System.out.println(deserialized);
}
}
}
This program prints the following:
[Hello, , World!]
This was kind of a silly example, though, because you would never serialize a list of strings like this — instead, you would probably write the strings to a regular text file with one line per string.
The Java Object Serialization Exercise on the next page has a slightly more realistic example for you to try out.
SOLUTION:
- To save its contents to file.
- To send it over a network.